Contents
SET FILE NAMES ACCORDING TO FOLDER NAME
back to top
myGroups = {'\EPCs\Group 1';...
'\EPCs\Group 2';...
'\MEPPs\Group 1';...
'\MEPPs\Group 2'};
for n = 1:size(myGroups,1)
currentFolder = pwd;
searchFolder = [pwd, myGroups{n}];
searchDir = dir(searchFolder);
searchDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
Dircount = 1;
for k = 1:length(searchDir)
if strcmpi(searchDir(k).name,'.') || strcmpi(searchDir(k).name,'..') || ~searchDir(k).isdir
continue;
else
searchDirtemp(Dircount,1) = searchDir(k);
Dircount = Dircount +1;
end
end
searchDir = searchDirtemp;
clear searchDirtemp Dircount
for j = 1:length(searchDir)
filePath = [searchFolder, '\', searchDir(j).name];
fileDir = dir(filePath);
fileDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
filecount = 1;
for k = 1:length(fileDir)
if strcmpi(fileDir(k).name,'.') || strcmpi(fileDir(k).name,'..')
continue;
else
fileDirtemp(filecount,1) = fileDir(k);
filecount = filecount + 1;
end
end
fileDir = fileDirtemp;
clear fileDirtemp filecount
for k = 1:length(fileDir)
oldfileName = fileDir(k).name;
newName1 = searchDir(j).name;
newName2temp = oldfileName;
newName2ind = strfind(newName2temp,'_');
newName2 = newName2temp(newName2ind:end);
newfileName = [newName1, newName2];
if ~strcmp(newfileName,oldfileName)
movefile([filePath, '\', oldfileName], [filePath, '\', newfileName]);
end
end
end
end
REGENERATE AUTOEVENTSTATS FILE, SPECIFICALLY EVENTDATASTATS
back to top
clear all
myGroups = {'\EPCs\Group 1';...
'\EPCs\Group 2';...
'\MEPPs\Group 1';...
'\MEPPs\Group 2'};
for n = 1:size(myGroups,1)
currentFolder = pwd;
searchFolder = [pwd myGroups{n}];
searchDir = dir(searchFolder);
searchDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
Dircount = 1;
for k = 1:length(searchDir)
if strcmpi(searchDir(k).name,'.') || strcmpi(searchDir(k).name,'..') || ~searchDir(k).isdir
continue;
else
searchDirtemp(Dircount,1) = searchDir(k);
Dircount = Dircount +1;
end
end
searchDir = searchDirtemp;
clear searchDirtemp Dircount
for j = 1:length(searchDir)
fileDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
filecount = 1;
fileDir = dir([searchFolder, '\', searchDir(j).name]);
for k = 1:length(fileDir)
if strcmpi(fileDir(k).name,'.') || strcmpi(fileDir(k).name,'..')
continue;
else
fileDirtemp(filecount,1) = fileDir(k);
filecount = filecount + 1;
end
end
fileDir = fileDirtemp;
for k = 1:length(fileDir)
if ~isempty(strfind(fileDir(k).name,'_autoEventStats.mat'))
Eventsfile = fileDir(k).name;
cellnameidx = strfind(Eventsfile,'_autoEventStats.mat');
cellname = Eventsfile(1:cellnameidx(end)-1);
limitsCrop = [];
peakThreshold = [];
abfFile = [cellname,'.abf'];
movefile([searchFolder, '\', searchDir(j).name, '\', Eventsfile], pwd)
copyfile([searchFolder, '\', searchDir(j).name, '\', abfFile], pwd)
load(Eventsfile);
if exist('fileCorrupt','var')
movefile(Eventsfile, [searchFolder, '\', searchDir(j).name]);
clear fileCorrupt
delete(abfFile);
continue
end
[mycell si] = abfload(abfFile);
si = si/1e6;
mycell = myFilter(mycell);
if ~isempty(limitsCrop)
leftlimit = myEvents(1,1);
rightlimit = myEvents(3,end);
EventsRange = rightlimit - leftlimit;
if limitsCrop(2)-limitsCrop(1) < EventsRange
limitsCrop = [];
end
end
if isempty(limitsCrop)
y1 = mycell;
else
y1 = mycell(limitsCrop(1):limitsCrop(2));
end
[EventDataStats hfigStats] = getEventStats(myEvents,y1,myEventsFlag,si);
close(hfigStats)
save([cellname,'_','autoEventStats', '.mat'],...
'EventDataStats', 'limitsCrop', 'myEvents',...
'myEventsFlag', 'peakSet', 'si', 'peakThreshold');
movefile(Eventsfile, [searchFolder, '\', searchDir(j).name]);
delete(abfFile);
clear EventDataStats limitsCrop myEvents myEventsFlag peakSet si peakThreshold y1 mycell
end
end
end
clear fileDirtemp filecount
end
SEPERATE ALL FILES INTO RESPECTIVE GROUPS
back to top
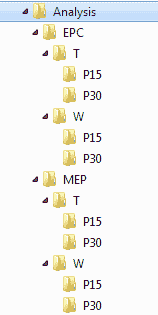
evalin('base','clear all; clc');
Analysisfolders = {'\Analysis\EPC\T\P15';
'\Analysis\EPC\T\P30';
'\Analysis\EPC\W\P15';
'\Analysis\EPC\W\P30';
'\Analysis\MEP\T\P15';
'\Analysis\MEP\T\P30';
'\Analysis\MEP\W\P15';
'\Analysis\MEP\W\P30';};
for k = 1:size(Analysisfolders,1)
mkdir([pwd, Analysisfolders{k}]);
end
myGroups = {'\EPCs\Group 1';...
'\EPCs\Group 2';...
'\MEPPs\Group 1';...
'\MEPPs\Group 2'};
for n = 1:size(myGroups,1)
currentFolder = pwd;
searchFolder = [pwd, myGroups{n}];
searchDir = dir(searchFolder);
searchDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
Dircount = 1;
for k = 1:length(searchDir)
if strcmpi(searchDir(k).name,'.') || strcmpi(searchDir(k).name,'..') || ~searchDir(k).isdir
continue;
else
searchDirtemp(Dircount,1) = searchDir(k);
Dircount = Dircount +1;
end
end
searchDir = searchDirtemp;
clear searchDirtemp Dircount
if ~isempty(strfind(myGroups{n},'EPCs'))
recordType = 'EPCs';
else
recordType = 'MEPPs';
end
for j = 1:length(searchDir)
searchfolderName = searchDir(j).name;
if strcmp(searchfolderName(1),'T')
recordGene = 'T';
elseif strcmp(searchfolderName(1),'W')
recordGene = 'W';
end
if ~isempty(strfind(searchfolderName,'P15')) || ~isempty(strfind(searchfolderName,'P1'))
recordAge = 'P15';
elseif ~isempty(strfind(searchfolderName,'P30')) || ~isempty(strfind(searchfolderName,'P3'))
recordAge = 'P30';
end
folderStringBuild = [recordType,recordGene,recordAge];
analysisString = {['EPCs','T','P15'];
['EPCs','T','P30'];
['EPCs','W','P15'];
['EPCs','W','P30'];
['MEPPs','T','P15'];
['MEPPs','T','P30'];
['MEPPs','W','P15'];
['MEPPs','W','P30']};
for k = 1:size(analysisString,1)
if strcmp(folderStringBuild,analysisString{k})
analysisPath = Analysisfolders{k};
break;
end
end
fileDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
filecount = 1;
searchPath = [searchFolder, '\', searchDir(j).name];
fileDir = dir(searchPath);
for k = 1:length(fileDir)
if strcmpi(fileDir(k).name,'.') || strcmpi(fileDir(k).name,'..')
continue;
else
fileDirtemp(filecount,1) = fileDir(k);
filecount = filecount + 1;
end
end
fileDir = fileDirtemp;
for k = 1:length(fileDir)
if ~isempty(strfind(fileDir(k).name,'autoEventStats.mat'))
Eventsfile = fileDir(k).name;
load([searchPath, '\', Eventsfile]);
if exist('fileCorrupt','var');
clear fileCorrupt
continue;
else
copyfile([searchPath, '\', Eventsfile], [pwd, analysisPath])
end
end
end
end
end
SEARCH THE ANALYSIS FOLDER DATA FOR ERRORS
back to top
evalin('base','clear all; clc');
rawDataPaths
Analysisfolders = {'\Analysis\EPC\T\P15';
'\Analysis\EPC\T\P30';
'\Analysis\EPC\W\P15';
'\Analysis\EPC\W\P30';
'\Analysis\MEP\T\P15';
'\Analysis\MEP\T\P30';
'\Analysis\MEP\W\P15';
'\Analysis\MEP\W\P30';};
for n = 1:size(Analysisfolders,1)
searchFolder = [pwd, Analysisfolders{n}];
fileDir = dir(searchFolder);
fileDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
filecount = 1;
for k = 1:length(fileDir)
if strcmpi(fileDir(k).name,'.') || strcmpi(fileDir(k).name,'..')
continue;
else
fileDirtemp(filecount,1) = fileDir(k);
filecount = filecount +1;
end
end
fileDir = fileDirtemp;
clear fileDirtemp filecount
fid = fopen(['Check_Events_Group_', num2str(n),'.txt'],'w');
myheader = 'File_Name \t Corrupt \t Events \t Flags \t Percent_Flags \t Re-analyze \r\n\n';
fprintf(fid, myheader);
hplot = figure('numbertitle','off');
for j = 1:length(fileDir)
if ~isempty(strfind(fileDir(j).name,'autoEventStats.mat'))
Eventsfile = fileDir(j).name;
cellnameidx = strfind(Eventsfile,'_autoEventStats.mat');
cellname = Eventsfile(1:cellnameidx(end)-1);
abfFile = [cellname,'.abf'];
load([searchFolder,'\',Eventsfile]);
if exist('fileCorrupt','var');
clear fileCorrupt
fprintf(fid,'%s \t', Eventsfile);
fprintf(fid,'%d \t %d \t %d \t %d \t %d \r\n', [1 NaN NaN NaN NaN]);
disp(['File Corrupt ', Eventsfile]);
continue;
end
[mycell si] = abfload(abfFile);
si = si/1e6;
mycell = myFilter(mycell);
if isempty(limitsCrop)
y1 = mycell;
else
y1 = mycell(limitsCrop(1):limitsCrop(2));
end
Eventsfile_print = regexprep(Eventsfile,'_','\\_');
set(hplot, 'Name', Eventsfile);
plot(y1,'k');
title({['Events = ', num2str(size(myEvents,1))]; Eventsfile_print});
hold on;
for k = 1:size(myEvents,1)
if myEventsFlag(k) == 0
plot(myEvents(k,1):myEvents(k,3),y1(myEvents(k,1):myEvents(k,3)),'g')
else
plot(myEvents(k,1):myEvents(k,3),y1(myEvents(k,1):myEvents(k,3)),'r')
end
end
hold off;
choice = questdlg('Are the Events Properly Aligned?', ...
'Event Detection Feedback', ...
'No','Yes','Yes');
switch choice
case 'No'
reAnalyze = 1;
case 'Yes'
reAnalyze = 0;
case ''
fclose(fid);
return
end
A = size(myEventsFlag,1);
B = sum(myEventsFlag);
C = B/A;
fprintf(fid,'%s \t', Eventsfile);
fprintf(fid,'%d \t %d \t %d \t %d \t %d \r\n', [0 A B C reAnalyze]);
end
end
fclose(fid);
end
USE ANALYSIS FOLDER TO GENERATE GROUP STATISTICS FOR EXPORT
back to top
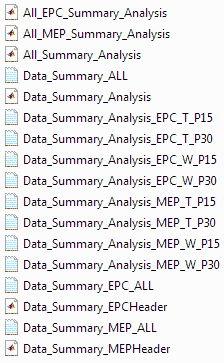

evalin('base','clear all; clc');
rawDataPaths
Analysisfolders = {'\Analysis\EPC\T\P15';
'\Analysis\EPC\T\P30';
'\Analysis\EPC\W\P15';
'\Analysis\EPC\W\P30';
'\Analysis\MEP\T\P15';
'\Analysis\MEP\T\P30';
'\Analysis\MEP\W\P15';
'\Analysis\MEP\W\P30';};
nameAnalysisfolders = regexprep(Analysisfolders, '\', '_');
SummaryAnalysisPath = [pwd '\Summary_Analysis'];
mkdir(SummaryAnalysisPath);
animalcountAll = 1;
cellcountAll = 1;
animalcountEPCAll = 1;
cellcountEPCAll = 1;
animalcountMEPAll = 1;
cellcountMEPAll = 1;
AllmyData = [];
AllmyEPCData = [];
AllmyMEPData = [];
for n = 1:size(Analysisfolders,1);
searchPath = [pwd, Analysisfolders{n}];
fileDir = dir(searchPath);
fileDirtemp = struct('name',{}, 'date',{}, 'bytes',{}, 'isdir',{}, 'datenum',{});
filecount = 1;
for k = 1:length(fileDir)
if strcmpi(fileDir(k).name,'.') || strcmpi(fileDir(k).name,'..')
continue;
else
fileDirtemp(filecount,1) = fileDir(k);
filecount = filecount + 1;
end
end
fileDir = fileDirtemp;
clear fileDirtemp filecount
cellcount = 1;
animalcount = 1;
fileName_string = {};
events_string = {};
myDataSummary = [];
myDataSummaryAll = [];
myDataSummaryEPCAll = [];
myDataSummaryMEPAll = [];
for k = 1:length(fileDir)
if k > 1
fileName1 = fileDir(k-1).name;
fileName2 = fileDir(k).name;
token1 = strfind(fileName1,'_');
animalName1 = fileName(1:token1(1)-1);
token2 =strfind(fileName2,'_');
animalName2 = fileName2(1:token2(1)-1);
if ~strcmp(animalName1,animalName2)
animalcount = animalcount+1;
animalcountAll = animalcountAll +1;
end
if ~strcmp(animalName1,animalName2) && n <= 4
animalcountEPCAll = animalcountEPCAll +1;
end
if ~strcmp(animalName1,animalName2) && n >= 5
animalcountMEPAll = animalcountMEPAll +1;
end
end
fileName = fileDir(k).name;
load([searchPath, '\', fileName]);
if exist('fileCorrupt','var');
clear fileCorrupt
continue;
end
if ~exist('EventDataStats','var') || ~exist('si','var')...
|| ~exist('peakSet','var') || ~exist('myEventsFlag','var')
continue;
end
mytime = myEvents(:,2)*si;
if size(EventDataStats,1) ~= size(mytime,1)
mytimetemp = ones(size(EventDataStats,1),1);
mytimecount = 1;
for j = 1:length(mytime)
if myEventsFlag(j) == 1
continue
else
mytimetemp(mytimecount) = mytime(j);
mytimecount = mytimecount +1;
end
end
mytime = mytimetemp;
end
rateF = nan(size(mytime));
for countF = 1:length(myEventsFlag)
if myEventsFlag(countF) == 0
startF = myEvents(countF,1);
break
end
end
for countF = length(myEventsFlag):-1:1
if myEventsFlag(countF) == 0
endF = myEvents(countF,3);
break
end
end
timeF = endF - startF;
timeF = timeF*si;
eventsF = sum(~myEventsFlag);
rateF(1) = eventsF / timeF;
iPitemp = nan(size(mytime));
iPi = diff(mytime);
iPitemp(1:length(iPi)) = iPi;
iPi = iPitemp;
fileName_print = regexprep(fileName,'.mat','');
fileName_string_temp = repmat(fileName_print, size(EventDataStats,1),1);
fileName_string_temp = cellstr(fileName_string_temp);
fileName_string = [fileName_string; fileName_string_temp];
events_string_temp = 1:size(EventDataStats,1);
events_string_temp = events_string_temp(:);
events_string_temp = num2str(events_string_temp);
events_string_temp = cellstr(events_string_temp);
events_string = [events_string;events_string_temp];
animalArray = ones(size(EventDataStats,1),1)*animalcount;
cellsArray = ones(size(EventDataStats,1),1)*cellcount;
animalArrayAll = ones(size(EventDataStats,1),1)*animalcountAll;
cellsArrayAll = ones(size(EventDataStats,1),1)*cellcountAll;
if n <= 4
animalArrayEPCAll = ones(size(EventDataStats,1),1)*animalcountEPCAll;
cellsArrayEPCAll = ones(size(EventDataStats,1),1)*cellcountEPCAll;
else
animalArrayMEPAll = ones(size(EventDataStats,1),1)*animalcountMEPAll;
cellsArrayMEPAll = ones(size(EventDataStats,1),1)*cellcountMEPAll;
end
groupArray = ones(size(EventDataStats,1),1)*n;
for p = 1:numel(EventDataStats)
if EventDataStats(p) < 0
EventDataStats(p) = NaN;
end
end
for p = 1:size(EventDataStats,1)
if EventDataStats(p,2) > 0.1
EventDataStats(p,2) = NaN;
end
if EventDataStats(p,3) > 0.1
EventDataStats(p,3) = NaN;
end
end
cellnameidx = strfind(fileName,'_autoEventStats.mat');
cellname = fileName(1:cellnameidx(end)-1);
abfFile = [cellname,'.abf'];
myfileN = abfFile;
fid = fopen(myfileN,'r','ieee-le');
sz=1;
numType = 'float';
myGainfileoffset = 268;
fseek(fid,myGainfileoffset,'bof');
cellGain = fread(fid,sz,numType);
fclose(fid);
EventDataStats(:,1) = EventDataStats(:,1)/cellGain;
EventDataStats(:,4) = EventDataStats(:,4)/cellGain;
myDataSummarytemp = [groupArray animalArray cellsArray EventDataStats iPi rateF mytime];
myDataSummaryAlltemp = [groupArray animalArrayAll cellsArrayAll EventDataStats iPi rateF mytime];
myDataSummary = [myDataSummary; myDataSummarytemp];
myDataSummaryAll = [myDataSummaryAll; myDataSummaryAlltemp];
cellcount = cellcount +1;
cellcountAll = cellcountAll +1;
if n <= 4
myDataSummaryEPCAlltemp = [groupArray animalArrayEPCAll cellsArrayEPCAll EventDataStats iPi rateF mytime];
myDataSummaryEPCAll = [myDataSummaryEPCAll; myDataSummaryEPCAlltemp];
cellcountEPCAll = cellcountEPCAll +1;
else
myDataSummaryMEPAlltemp = [groupArray animalArrayMEPAll cellsArrayMEPAll EventDataStats iPi rateF mytime];
myDataSummaryMEPAll = [myDataSummaryMEPAll; myDataSummaryMEPAlltemp];
cellcountMEPAll = cellcountMEPAll +1;
end
clear EventDataStats limitsCrop myEvents myEventsFlag peakSet peakThreshold si
end
eval(['DataSummary', nameAnalysisfolders{n}, '= myDataSummary;']);
EventGeneAge = {['EPC','\t', 'TWI','\t', 'P15','\t'];
['EPC','\t', 'TWI','\t', 'P30','\t'];
['EPC','\t', 'WT ','\t', 'P15','\t'];
['EPC','\t', 'WT ','\t', 'P30','\t'];
['MEP','\t', 'TWI','\t', 'P15','\t'];
['MEP','\t', 'TWI','\t', 'P30','\t'];
['MEP','\t', 'WT ','\t', 'P15','\t'];
['MEP','\t', 'WT ','\t', 'P30','\t']};
if n <= 4
fid = fopen(['Data_Summary', nameAnalysisfolders{n}, '.txt'], 'w');
fprintf(fid, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(nA) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(nA*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \t Event \t File Name \r\n\n');
for p = 1:size(myDataSummary,1)
fprintf(fid, EventGeneAge{n});
fprintf(fid, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t', myDataSummary(p,:));
fprintf(fid, [events_string{p}, ' \t ', fileName_string{p}, ' \r']);
end
else
fid = fopen(['Data_Summary', nameAnalysisfolders{n}, '.txt'], 'w');
fprintf(fid, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(mV) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(mV*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \t Event \t File Name \r\n\n');
for p = 1:size(myDataSummary,1)
fprintf(fid, EventGeneAge{n});
fprintf(fid, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t', myDataSummary(p,:));
fprintf(fid, [events_string{p}, ' \t ', fileName_string{p}, ' \r']);
end
end
fclose(fid);
movefile(['*',nameAnalysisfolders{n}, '.txt'], SummaryAnalysisPath);
clear myDataSummary
if n == 1
fidAll = fopen(['Data_Summary_ALL', '.txt'], 'w');
fprintf(fidAll, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(nA) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(nA*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \r\n\n');
for p = 1:size(myDataSummaryAll,1)
fprintf(fidAll, EventGeneAge{n});
fprintf(fidAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryAll(p,:));
end
elseif n == 5
fprintf(fidAll, ' \r\n\n');
fprintf(fidAll, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(mV) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(mV*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \r\n\n');
for p = 1:size(myDataSummaryAll,1)
fprintf(fidAll, EventGeneAge{n});
fprintf(fidAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryAll(p,:));
end
else
for p = 1:size(myDataSummaryAll,1)
fprintf(fidAll, EventGeneAge{n});
fprintf(fidAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryAll(p,:));
end
end
AllmyData = [AllmyData; myDataSummaryAll];
animalcountAll = animalcountAll +1;
clear myDataSummaryAll
if n <= 4
if n == 1
fidEPCAll = fopen(['Data_Summary_EPC_ALL', '.txt'], 'w');
fprintf(fidEPCAll, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(nA) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(nA*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \r\n\n');
for p = 1:size(myDataSummaryEPCAll,1)
fprintf(fidEPCAll, EventGeneAge{n});
fprintf(fidEPCAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryEPCAll(p,:));
end
else
for p = 1:size(myDataSummaryEPCAll,1)
fprintf(fidEPCAll, EventGeneAge{n});
fprintf(fidEPCAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryEPCAll(p,:));
end
end
AllmyEPCData = [AllmyEPCData; myDataSummaryEPCAll];
animalcountEPCAll = animalcountEPCAll +1;
clear myDataSummaryEPCAll
end
if n >= 5
if n == 5
fidMEPAll = fopen(['Data_Summary_MEP_ALL', '.txt'], 'w');
fprintf(fidMEPAll, 'Event \t Gene \t Age \t Group \t Animal \t Cell \t Amplitude(mV) \t 1/Tau-Rise(sec) \t 1/Tau-Decay(sec) \t AreaUnderCurve(mV*sec) \t Rsquare(rise) \t Rsquare(decay) \t InterPeakInterval(sec) \t Frequency(events/sec) \t time(sec) \r\n\n');
for p = 1:size(myDataSummaryMEPAll,1)
fprintf(fidMEPAll, EventGeneAge{n});
fprintf(fidMEPAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryMEPAll(p,:));
end
else
for p = 1:size(myDataSummaryMEPAll,1)
fprintf(fidMEPAll, EventGeneAge{n});
fprintf(fidMEPAll, '%10d \t %10d \t %10d \t %10.5f \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \t %10.5e \r', myDataSummaryMEPAll(p,:));
end
end
AllmyMEPData = [AllmyMEPData; myDataSummaryMEPAll];
animalcountMEPAll = animalcountMEPAll +1;
clear myDataSummaryEPCAll
end
end
fclose(fidAll);
fclose(fidEPCAll);
fclose(fidMEPAll);
movefile('Data_Summary_ALL.txt', SummaryAnalysisPath);
movefile('Data_Summary_EPC_ALL.txt', SummaryAnalysisPath);
movefile('Data_Summary_MEP_ALL.txt', SummaryAnalysisPath);
save('Data_Summary_Analysis','DataSummary_Analysis*');
movefile('Data_Summary_Analysis.mat', SummaryAnalysisPath);
save('All_Summary_Analysis','AllmyData');
movefile('All_Summary_Analysis.mat', SummaryAnalysisPath);
save('All_EPC_Summary_Analysis','AllmyEPCData');
movefile('All_EPC_Summary_Analysis.mat', SummaryAnalysisPath);
save('All_MEP_Summary_Analysis','AllmyMEPData');
movefile('All_MEP_Summary_Analysis.mat', SummaryAnalysisPath);
EPCheader = {'Group'; 'Animal'; 'Cell'; 'Amplitude(nA)'; '1/Tau-Rise(sec)'; '1/Tau-Decay(sec)'; 'AreaUnderCurve(nA*sec)'; 'Rsquare(rise)'; 'Rsquare(decay)'; 'InterPeakInterval(sec)'; 'Frequency(events/sec)'; 'time(sec)'; 'Events'; 'File Name'};
MEPheader = {'Group'; 'Animal'; 'Cell'; 'Amplitude(mV)'; '1/Tau-Rise(sec)'; '1/Tau-Decay(sec)'; 'AreaUnderCurve(mV*sec)'; 'Rsquare(rise)'; 'Rsquare(decay)'; 'InterPeakInterval(sec)'; 'Frequency(events/sec)'; 'time(sec)'; 'Events'; 'File Name'};
save('Data_Summary_EPCHeader', 'EPCheader');
save('Data_Summary_MEPHeader', 'MEPheader');
movefile('Data_Summary_EPCHeader.mat', SummaryAnalysisPath);
movefile('Data_Summary_MEPHeader.mat', SummaryAnalysisPath);