Contents
INITIALIZE GUI
back to top
function varargout = NMJ_GUI(varargin)
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @NMJ_GUI_OpeningFcn, ...
'gui_OutputFcn', @NMJ_GUI_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
function NMJ_GUI_OpeningFcn(hObject, eventdata, handles, varargin)
handles.output = hObject;
guidata(hObject, handles);
addpath(genpath([cd,'\control']));
addpath(genpath([cd,'\iso2mesh']));
addpath(genpath([cd,'\images']));
evalin('base','loadmyimages')
MyI = evalin('base','MyI');
MyIfilt = myfilter(MyI);
assignin('base','MyIfilt',MyIfilt);
axes(handles.axes1);
imagesc(MyI(:,:,1));colormap(pink);axis off;
set(handles.axes1,'visible','off')
axes(handles.axes2);
axis off;
set(handles.axes2,'visible','off')
axes(handles.axes3);
axis off;
set(handles.axes3,'visible','off')
temp = 1:size(MyI,3);
temp = temp';
A = cellstr(num2str(temp));
MyString = A;
set(handles.listbox1,'String',MyString);
assignin('base','MyString',MyString);
mymax = 1;
mymin = 0;
mystep = [.01,.1];
set(handles.slider2,'Min',mymin);
set(handles.slider2,'Max',mymax);
set(handles.slider2,'SliderStep', mystep);
set(handles.slider2,'Value',1);
set(handles.slider2,'interruptible','off');
slider2pos = get(handles.slider2,'Value');
set(handles.text6,'String',num2str(slider2pos));
applycrop = 0;
Roiring = 0;
BwMask = [];
xi = 0;
yi = 0;
gofilt = 0;
ThreshLevel = [];
assignin('base','applycrop',applycrop);
assignin('base','Roiring',Roiring);
assignin('base','BwMask',BwMask);
assignin('base','xi',xi);
assignin('base','yi',yi);
assignin('base','gofilt',gofilt);
assignin('base','ThreshLevel',ThreshLevel);
function varargout = NMJ_GUI_OutputFcn(hObject, eventdata, handles)
varargout{1} = handles.output;
function listbox1_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function listbox1_KeyPressFcn(hObject, eventdata, handles)
function slider2_CreateFcn(hObject, eventdata, handles)
if isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor',[.9 .9 .9]);
end
FILTER CHECKBOX
back to top
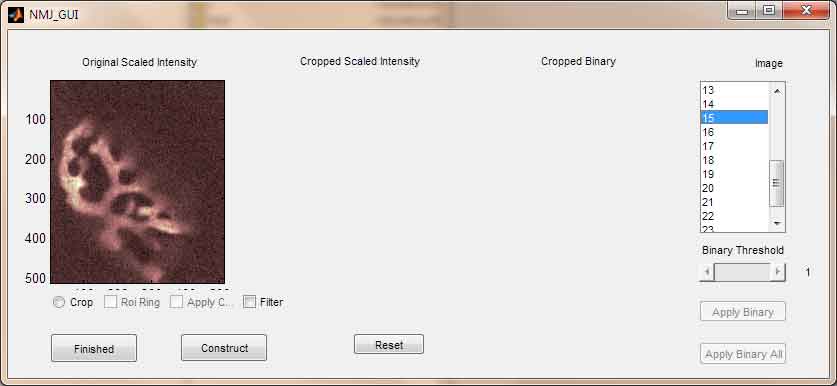
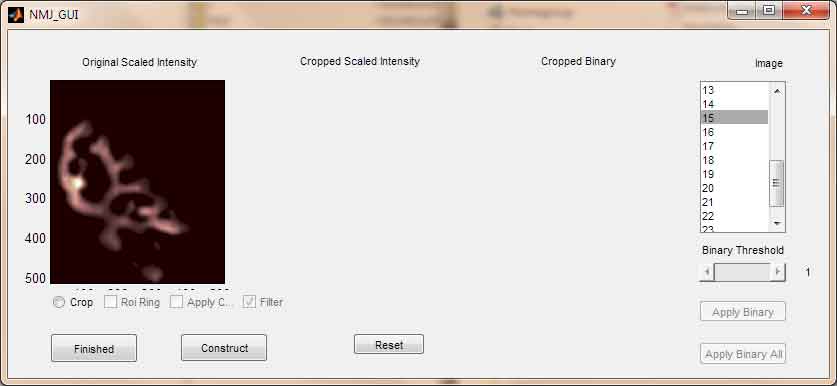
function checkbox3_Callback(hObject, eventdata, handles)
set(handles.checkbox3,'enable','off');
assignin('base','gofilt',1);
evalin('base','clear MyI; MyI = MyIfilt;');
MyI = evalin('base','MyI');
contentL = get(handles.listbox1,'Value');
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
set(handles.radiobutton1,'enable','on');
IMAGE LISTBOX
back to top
function listbox1_Callback(hObject, eventdata, handles)
MyI = evalin('base','MyI');
contentL = get(handles.listbox1,'Value');
applycrop = evalin('base','applycrop');
BwMask = evalin('base','BwMask');
Roiring = evalin('base','Roiring');
if applycrop == 1 && ~isempty(BwMask) && Roiring == 1
xi = evalin('base','xi');
yi = evalin('base','yi');
MyIcropsc = evalin('base','MyIcropsc');
MyIBw = evalin('base','MyIBw');
ThreshLevel = evalin('base','ThreshLevel');
ThreshLevel = ThreshLevel(contentL);
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
hold on;
plot(xi,yi);
hold off;
axes(handles.axes2);
set(handles.axes2,'visible','off')
imagesc(MyIcropsc(:,:,contentL));colormap(pink);
axes(handles.axes3);
set(handles.axes3,'visible','off')
imagesc(MyIBw(:,:,contentL));colormap(pink);
set(handles.slider2,'Value',ThreshLevel);
set(handles.text6,'String',num2str(ThreshLevel));
elseif applycrop == 1 && ~isempty(BwMask) && Roiring == 0
MyIcropsc = evalin('base','MyIcropsc');
MyIBw = evalin('base','MyIBw');
ThreshLevel = evalin('base','ThreshLevel');
ThreshLevel = ThreshLevel(contentL);
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
axes(handles.axes2);
set(handles.axes2,'visible','off')
imagesc(MyIcropsc(:,:,contentL));colormap(pink);
axes(handles.axes3);
set(handles.axes3,'visible','off')
imagesc(MyIBw(:,:,contentL));colormap(pink);
set(handles.slider2,'Value',ThreshLevel);
set(handles.text6,'String',num2str(ThreshLevel));
elseif applycrop == 0 && ~isempty(BwMask) && Roiring == 1
xi = evalin('base','xi');
yi = evalin('base','yi');
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
hold on;
plot(xi,yi);
hold off;
elseif applycrop == 0 && isempty(BwMask) && Roiring == 1
xi = evalin('base','xi');
yi = evalin('base','yi');
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
hold on;
plot(xi,yi);
hold off;
else
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,contentL));colormap(pink);
end
CROP BUTTON
back to top
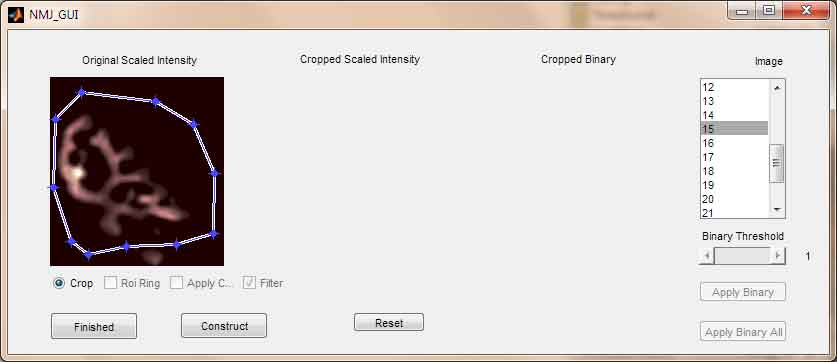
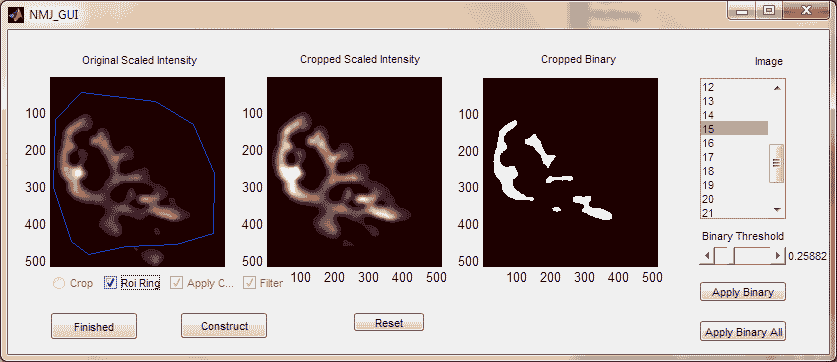
function radiobutton1_Callback(hObject, eventdata, handles)
MyI = evalin('base','MyI');
contentL = get(handles.listbox1,'Value');
axes(handles.axes1);
set(handles.axes1,'visible','off')
[BwMask, xi, yi] = roipoly;
assignin('base','xi',xi);
assignin('base','yi',yi);
assignin('base','BwMask',BwMask);
set(handles.radiobutton1,'Value',0);
set(handles.radiobutton1,'enable','off');
set(handles.checkbox3,'enable','off');
disp('Cropped');
for k = 1:size(MyI,3);
MyIcrop(:,:,k) = double(MyI(:,:,k)).*double(BwMask);
end
MyIcrop = uint8(MyIcrop);
assignin('base','MyIcrop',MyIcrop);
MyIcropsc = zeros(size(MyIcrop),'uint8');
MyIcropscd = double(MyIcropsc(:,:,1));
for k = 1:size(MyIcrop,3);
MyIcropscd(:,:,1) = double(MyIcrop(:,:,k)) - min(min(double(MyIcrop(:,:,k))));
MyIcropsc(:,:,k) = uint8(MyIcropscd * 255 / (max(MyIcropscd(:))));
end
clear MyIcropscd;
assignin('base','MyIcropsc',MyIcropsc);
MyIBw = logical(MyIcropsc);
se = strel('disk',3);
ThreshLevel = evalin('base','ThreshLevel');
for k = 1:size(MyIBw,3);
ThreshLevel(k) = graythresh(MyIcropsc(:,:,k));
MyIBwtemp = im2bw(MyIcropsc(:,:,k),ThreshLevel(k));
MyIBwtemp = bwareaopen(MyIBwtemp,200);
MyIBw(:,:,k) = imclose(MyIBwtemp,se);
end
assignin('base','MyIBw',MyIBw);
assignin('base','ThreshLevel',ThreshLevel);
axes(handles.axes2);
set(handles.axes2,'visible','off')
imagesc(MyIcropsc(:,:,contentL));colormap(pink);
axes(handles.axes3);
set(handles.axes3,'visible','off')
imagesc(MyIBw(:,:,contentL));colormap(pink);
set(handles.checkbox1,'enable','on');
set(handles.checkbox2,'enable','on');
set(handles.slider2,'enable','on');
myThreshLevel = ThreshLevel(contentL);
set(handles.slider2,'Value',myThreshLevel);
set(handles.text6,'String',num2str(myThreshLevel));
set(handles.togglebutton3,'enable','on');
set(handles.togglebutton4,'enable','on');
set(handles.checkbox2,'value',1);
assignin('base','applycrop',1);
set(handles.checkbox2,'enable','off');
ROI RING CHECKBOX
back to top
function checkbox1_Callback(hObject, eventdata, handles)
Roiring = get(handles.checkbox1,'Value');
assignin('base','Roiring',Roiring);
if Roiring == 0;
axes(handles.axes1);
set(handles.axes1,'visible','off')
MyI = evalin('base','MyI');
contentL = get(handles.listbox1,'Value');
imagesc(MyI(:,:,contentL));colormap(pink);
else
axes(handles.axes1);
set(handles.axes1,'visible','off')
MyI = evalin('base','MyI');
contentL = get(handles.listbox1,'Value');
imagesc(MyI(:,:,contentL));colormap(pink);
hold on;
xi = evalin('base', 'xi');
yi = evalin('base', 'yi');
plot(xi,yi,'b-');
hold off;
end
APPLY CROP CHECKBOX
back to top
function checkbox2_Callback(hObject, eventdata, handles)
applycrop = get(handles.checkbox2,'Value');
assignin('base','applycrop',applycrop);
set(handles.checkbox2,'enable','off');
BINARY THRESHOLD
back to top
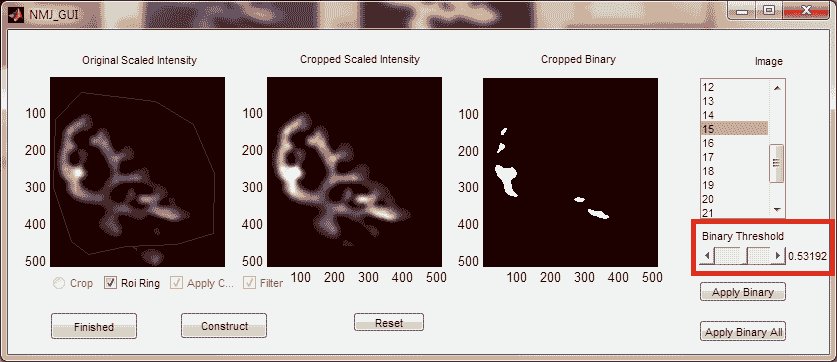
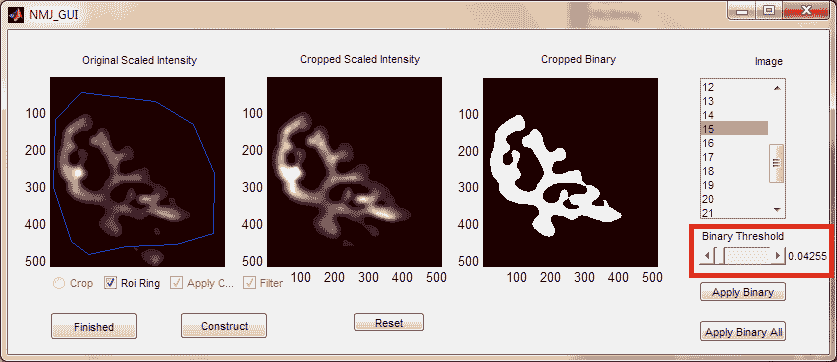
function slider2_Callback(hObject, eventdata, handles)
slider2pos = get(handles.slider2,'Value');
set(handles.text6,'String',num2str(slider2pos));
contentL = get(handles.listbox1,'Value');
assignin('base','contentL',contentL);
MyIcropsc = evalin('base','MyIcropsc(:,:,contentL)');
se = strel('disk',3);
ThreshLevel = slider2pos;
MyIBwtemp = im2bw(MyIcropsc,ThreshLevel);
MyIBwtemp = bwareaopen(MyIBwtemp,200);
MyIBw = imclose(MyIBwtemp,se);
axes(handles.axes3);
set(handles.axes3,'visible','off')
imagesc(MyIBw);colormap(pink);
APPLY THRESHOLD
back to top
function togglebutton3_Callback(hObject, eventdata, handles)
slider2pos = get(handles.slider2,'Value');
contentL = get(handles.listbox1,'Value');
assignin('base','contentL',contentL);
MyIcropsc = evalin('base','MyIcropsc(:,:,contentL)');
se = strel('disk',3);
ThreshLevel = slider2pos;
MyIBwtemp = im2bw(MyIcropsc,ThreshLevel);
MyIBwtemp = bwareaopen(MyIBwtemp,200);
MyIBw = imclose(MyIBwtemp,se);
assignin('base','ThreshLeveltemp',ThreshLevel);
assignin('base','MyIBwtemp',MyIBw);
evalin('base','ThreshLevel(contentL) = ThreshLeveltemp;');
evalin('base', 'MyIBw(:,:,contentL) = MyIBwtemp;');
set(hObject,'Value',0);
APPLY ALL THRESHOLD
back to top
function togglebutton4_Callback(hObject, eventdata, handles)
slider2pos = get(handles.slider2,'Value');
contentL = get(handles.listbox1,'Value');
assignin('base','contentL',contentL);
MyIcropsc = evalin('base','MyIcropsc');
se = strel('disk',3);
ThreshLevel = slider2pos;
MyIBw = logical(MyIcropsc);
for k = 1:size(MyIcropsc,3);
MyIBwtemp = im2bw(MyIcropsc(:,:,k),ThreshLevel);
MyIBwtemp = bwareaopen(MyIBwtemp,200);
MyIBw(:,:,k) = imclose(MyIBwtemp,se);
end
ThreshLevel = ThreshLevel*ones(size(MyIBw,3),1);
assignin('base','ThreshLevel',ThreshLevel);
assignin('base','MyIBw',MyIBw);
set(hObject,'Value',0);
RESET BUTTON
back to top
function togglebutton1_Callback(hObject, eventdata, handles)
applycrop = 0;
Roiring = 0;
BwMask = [];
xi = 0;
yi = 0;
gofilt = 0;
ThreshLevel = [];
assignin('base','applycrop',applycrop);
assignin('base','Roiring',Roiring);
assignin('base','BwMask',BwMask);
assignin('base','xi',xi);
assignin('base','yi',yi);
assignin('base','gofilt',gofilt);
assignin('base','ThreshLevel',ThreshLevel);
evalin('base','clear MyI; MyI = I;');
MyI = evalin('base','MyI');
axes(handles.axes1);
set(handles.axes1,'visible','off')
imagesc(MyI(:,:,1));colormap(pink);axis off;
set(handles.listbox1,'Value',1);
axes(handles.axes2);
set(handles.axes2,'visible','off')
cla;
axis off;
axes(handles.axes3);
set(handles.axes3,'visible','off')
cla;
axis off;
set(handles.radiobutton1,'Value',0);
set(handles.checkbox1,'Value',0);
set(handles.checkbox2,'Value',0);
set(handles.checkbox3,'Value',0);
set(handles.checkbox1,'enable','off');
set(handles.checkbox2,'enable','off');
set(handles.slider2,'enable','off');
set(handles.togglebutton3,'enable','off');
set(handles.togglebutton4,'enable','off');
set(handles.checkbox3,'enable','on');
set(handles.radiobutton1,'enable','on');
set(hObject,'Value',0);
disp('reset')
FINISHED BUTTON
back to top
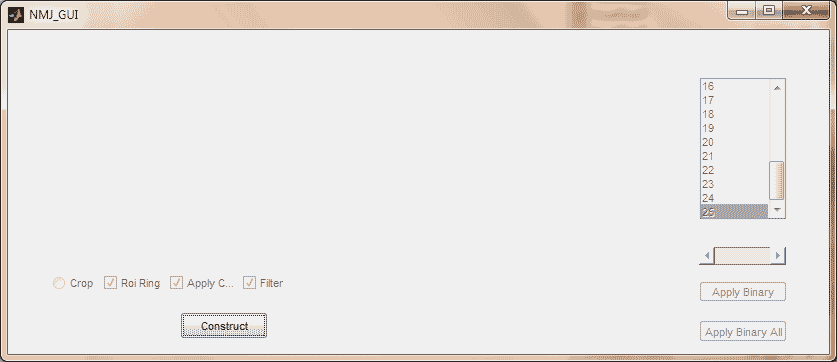
function pushbutton1_Callback(hObject, eventdata, handles)
set(handles.checkbox1,'enable','off');
set(handles.checkbox2,'enable','off');
set(handles.slider2,'enable','off');
set(handles.togglebutton3,'enable','off');
set(handles.togglebutton4,'enable','off');
set(handles.checkbox3,'enable','off');
set(handles.radiobutton1,'enable','off');
set(handles.listbox1,'enable','off');
set(handles.text1,'visible','off');
set(handles.text2,'visible','off');
set(handles.text3,'visible','off');
set(handles.text4,'visible','off');
set(handles.text5,'visible','off');
set(handles.text6,'visible','off');
set(handles.togglebutton1,'visible','off');
set(handles.pushbutton1,'visible','off');
set(handles.axes1,'visible','off')
axes(handles.axes1); cla;
set(handles.axes2,'visible','off')
axes(handles.axes2); cla;
set(handles.axes3,'visible','off')
axes(handles.axes3); cla;
evalin('base', 'clear BwMask I MyI MyIBw MyIBwtemp MyIcrop MyIfilt');
CONSTRUCT BUTTON
back to top
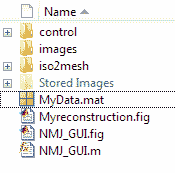
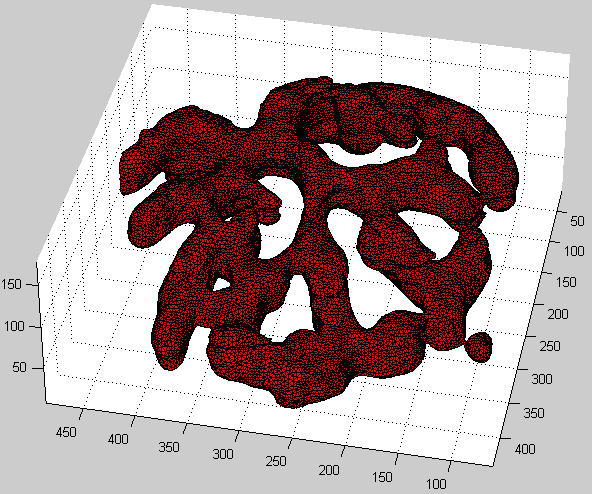
function pushbutton2_Callback(hObject, eventdata, handles)
o = 'This will take a while...';
assignin('base','o',o);
oo = 'Wait...';
assignin('base','oo',oo);
evalin('base', 'disp(o)');
evalin('base', 'Iinterp = myIinterp(MyIcropsc,ThreshLevel);' );
evalin('base', 'disp(oo)');
evalin('base', '[V,SA,h] = myIConstruct(Iinterp);' );